I was looking for ways to show periodically updating Python plots in WordPress and while there were a few libraries available they aren’t compatible with all themes.
You will need access to the server that your website is running to save the plot in HTML form, in this case, I was using Plotly and saving to the uploads folder of my server.
Simply start either a page or a post, select a block and search ‘HTML’ and select the ‘Custom HTML option’
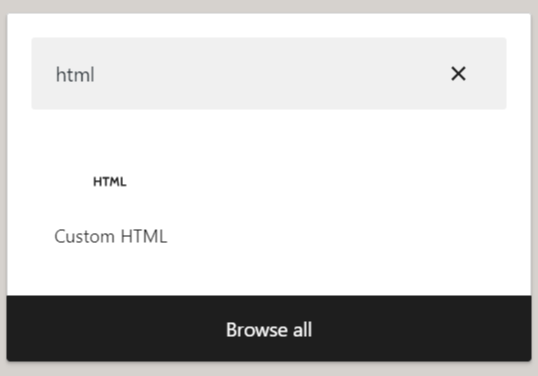
This section will take care of the formatting of your section
<div id="custom-html-container" style="background-color: rgb(214, 210, 206);">
<iframe id="custom-iframe" src="" width="100%" height="500px" frameborder="0"></iframe>
</div>
Next, we want to load a function that updates the custom HTML container
<script>
// Function to update the src attribute of the iframe
function updateIframeSrc(url) {
document.getElementById('custom-iframe').src = url;
}
// Function to fetch and update custom HTML content
function fetchCustomHTML() {
// update path to your file location
fetch('https://website.com.au/wp-content/custom_html/html_file.html')
.then(response => response.text())
.then(html => {
// Update the src attribute of the iframe
updateIframeSrc('https://website.com.au/wp-content/custom_html/html_file.html');
})
.catch(error => {
console.error('Error fetching custom HTML:', error);
});
}
call the function
// Call the function initially to load the custom HTML content
fetchCustomHTML();
finally, set the interval and close the script
// Refresh the custom HTML content every 10 seconds
setInterval(fetchCustomHTML, 10000); // Adjust the interval as needed
</script>
Complete code:
<div id="custom-html-container" style="background-color: rgb(214, 210, 206);">
<iframe id="custom-iframe" src="" width="100%" height="500px" frameborder="0"></iframe>
</div>
<script>
// Function to update the src attribute of the iframe
function updateIframeSrc(url) {
document.getElementById('custom-iframe').src = url;
}
// Function to fetch and update custom HTML content
function fetchCustomHTML() {
// update path to your file location
fetch('https://website.com.au/wp-content/custom_html/html_file.html')
.then(response => response.text())
.then(html => {
// Update the src attribute of the iframe
updateIframeSrc('https://website.com.au/wp-content/custom_html/html_file.html');
})
.catch(error => {
console.error('Error fetching custom HTML:', error);
});
}
// Call the function initially to load the custom HTML content
fetchCustomHTML();
// Refresh the custom HTML content every 10 seconds
setInterval(fetchCustomHTML, 10000); // Adjust the interval as needed
</script>
The below is loaded with a version of this code.
Leave a Reply